For people just learning how to code, there are several things that can be hard to get your head around at first, and there may be ways to address each quirk that haven't been exhausted yet. Let's dig in:
Code is sort of English but really isn't.
Many well-meaning developers seem to think that code is hard because it's not enough like English. That's not the central issue. English-like words in code are simply stand-ins for symbols. Using "start" and "end" isn't any clearer than using "{" and "}"; in fact, the latter is more clear because it's more concise, icon-like, and less open to interpretation or synonyms. When we speak a human language, we think in concepts, not precise words. The word "start" goes into a memory slot in your head that contains a soup of implications around that word. When it comes time to write code again you might easily type "begin" instead of "start", because in English, both words mean the same thing, and you'd be understood. The compiler, however, does not know that "start" is a word with meaning. As far as the compiler is concerned, "start" could just as well be "J3KS;7". Say "begin" and the robot doorman will simply shrug and say "error". "Start" is a kind of compromise. It's a word picked from many similar words to help you remember what to type, but it's still a magic incantation that must be recalled precisely.
There is so much googling.
So much. Far too much to memorize, partially because of the "not-English" problem. Once a beginner gets beyond the standard toolset of understanding loops, dictionaries and the like, they enter the world of libraries, frameworks, assorted functions. Beginners think coding is sitting at the glow of a simple terminal, austerely typing like Hemingway. But then the googling begins, and it never stops. Senior level developers google constantly.
- What's the name of a function that does this? (or the one I used to know but forgot the name of?)
- What library is it in?
- How does the syntax go?
- Has this problem I'm working on been solved by someone else?
Of these questions, the last one makes sense, but from a beginner's perspective the first three feel like failure. It's like trying to order a beer in Chinese, knowing that there's a word for bottle, but being unable to find "bottle" in your dictionary because the right function is called "orderChineseBeer" and it's hiding in a library called "ChinaTrip". You'll find it eventually by searching StackOverflow, but if you happen to misremember it months later as "askChinaTripBeer" you'll have to do that search again. Your IDE will not help unless you start by typing "order".
You also might want to rotate an image using a graphics library that you know has this function in it, but because you search for "rotate", you won't find "turn" or "transform" or "tweakyboi" or whatever the dev that put this library together thought was a good name at the time. These words have no meaning in themselves, and specific meanings of real words are not exclusive to those words alone.
Possible solutions:
- Ideally library docs would let you search function descriptions as well as names. Perhaps AI could be applied to this.
- To broaden a search, one could cross-reference function names and descriptions with a thesaurus database, so that a search that returned no results for "turn" might be broadened to show "rotate" and "transform".
- The above could apply to text completion while typing. Type "turn", IDE checks if "turn" exists in included libraries, and if not, suggests related function names that do exist.
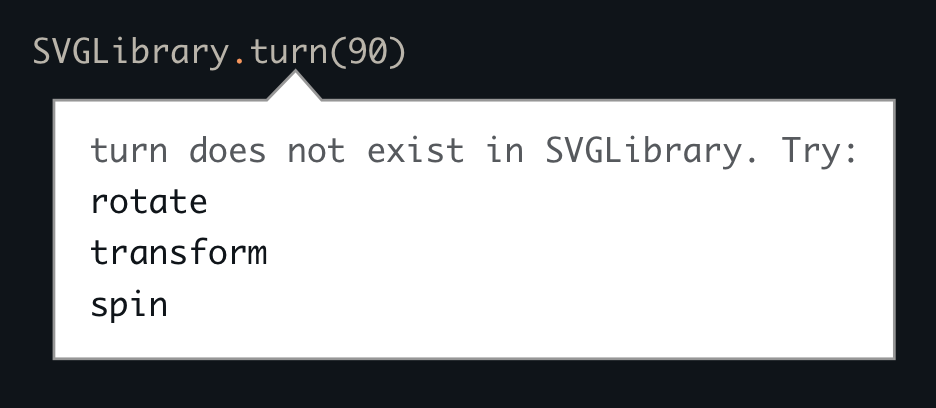
- If one found oneself repeatedly misremembering a function name, one could create an alias word to replace a really stupidly named function.
- As one uses an IDE, collect frequently used functions and libraries to improve suggestions.
- Bonus wish: let all code documentation include code examples, preferrably interactive. Codea for iPad is the best example of integrated documentation in an IDE that I've seen (plus some other very nice features), but even they might benefit from this.
Syntax is hilighted, but structure can be obscure.
Syntax hilighting is the equivalent of coloring all nouns in a paragraph red, all verbs green, all adjectives purple, and so on. This makes sense and helps a lot when you're focusing on constructing sentences. It doesn't tell you anything, however, about the big picture of what the code itself is doing.
Possible solutions:
- English literature has classic examples of various structures, as well as examples of literature considered "good". I've been searching, but have yet to find a resource for beginners that would showcase some classic basic structures to scaffold on, dissect them to show how and why they work and how to apply them in your own projects. There also seem to be a lot of people ready to point at certain code as "bad code" but not a whole lot of examples of what everyone agrees is "good code" for beginners to emulate. This might be a good thing to put together.
- Thematic hilighting in addition to syntax hilighting. This is a weird one that is perhaps more useful for analyzing code that someone else has made, or making broad brush "notes" on code you wrote that you need to revisit later. If you hate color, you're going to hate this. If you went to art school, are a bit dyslexic, or like organizing your files with color labels, this might be for you:
I experimented with color and language in a side project called RainbowEdit, which turned out to be useful for learning and analyzing new languages. So when I was considering code, I started another side project experiment called RainbowIDE which you can play with here. (works best with Chrome) All it does is accept pasted text files, and lets you color parts of the code as you like.
The central point is this: If you color parts of the code according to what they do instead of what they are, you start to see themes of meaning and overall structure. Themes can be all functions from a particular hardware library, or all functions that draw on the screen, or all functions that handle game logic. What you pick and what colors or text styles make sense to you are up to you.
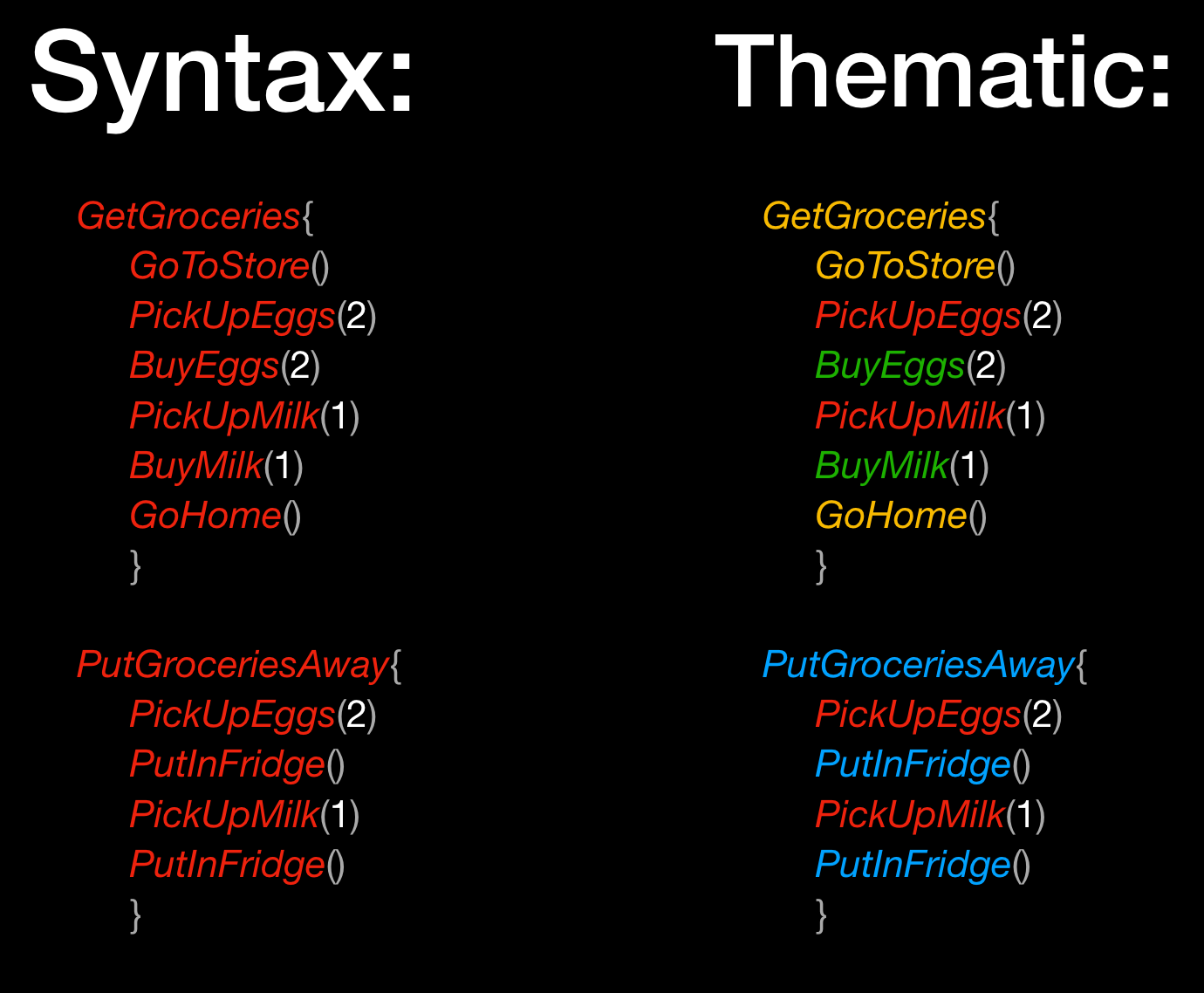
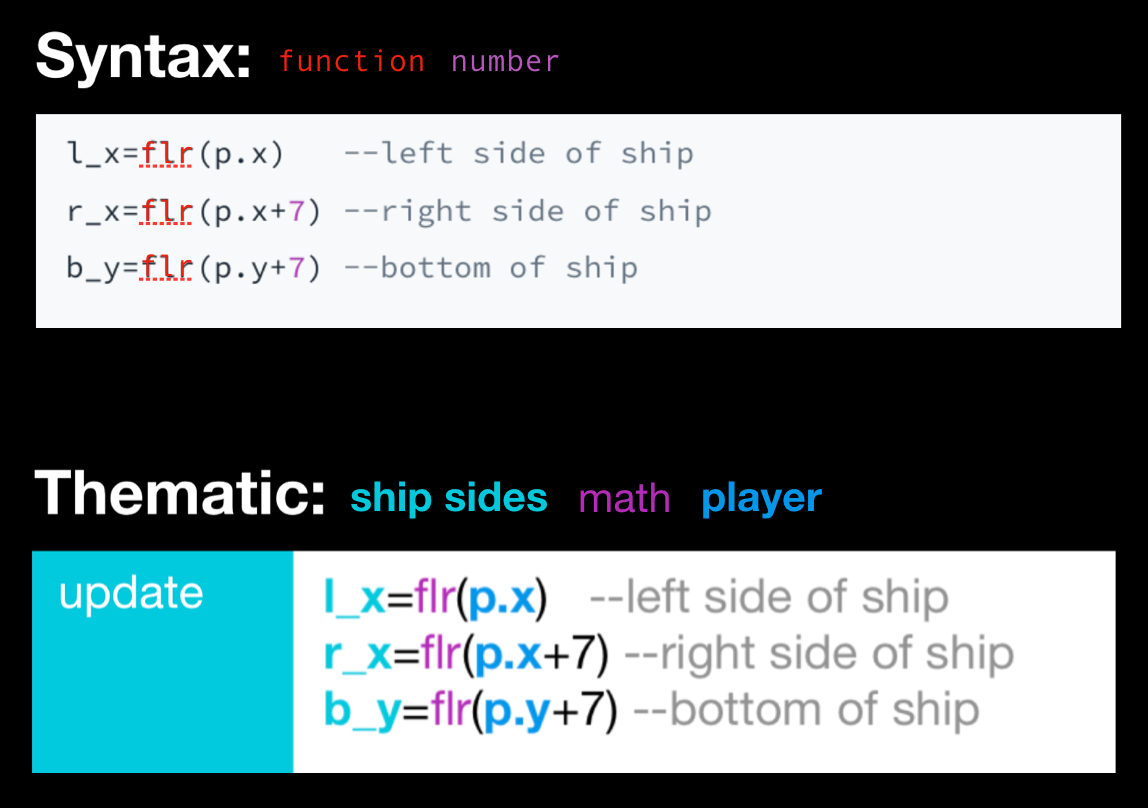
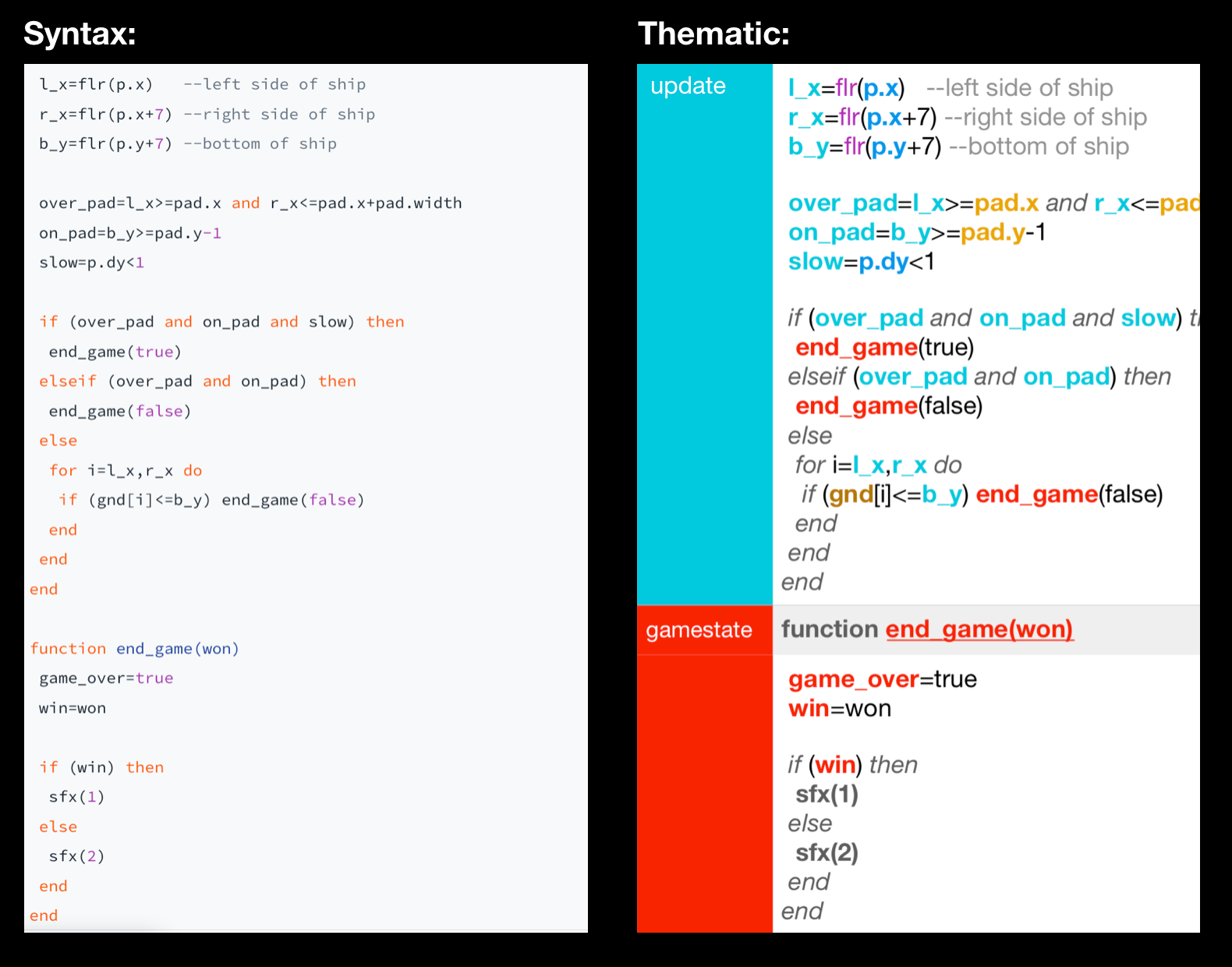
I've generally started with a monochrome greyscale for everything at first (obscuring end of line semicolons and other non-human-relevant elements by making them darker) . The end result can look something like this:
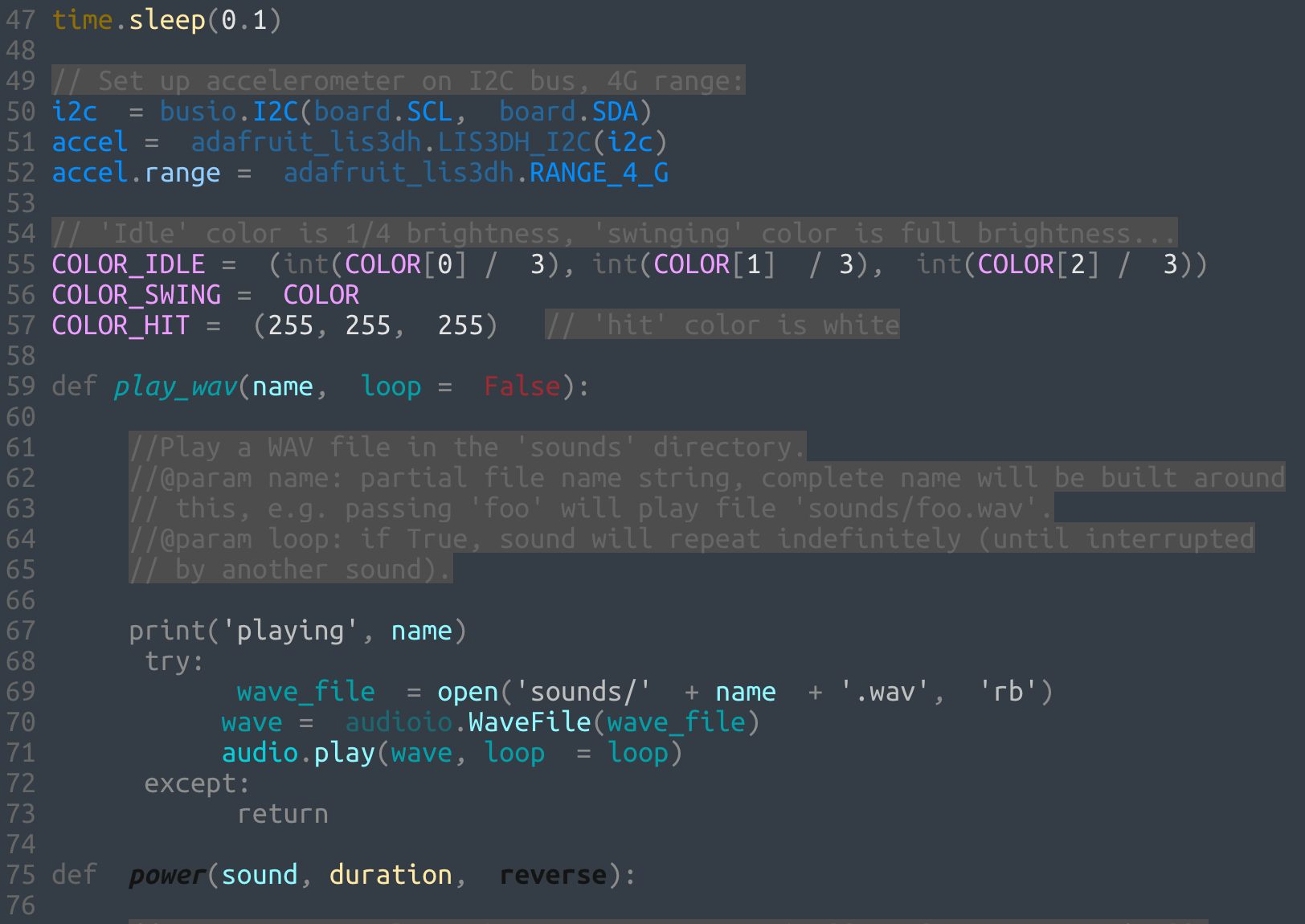
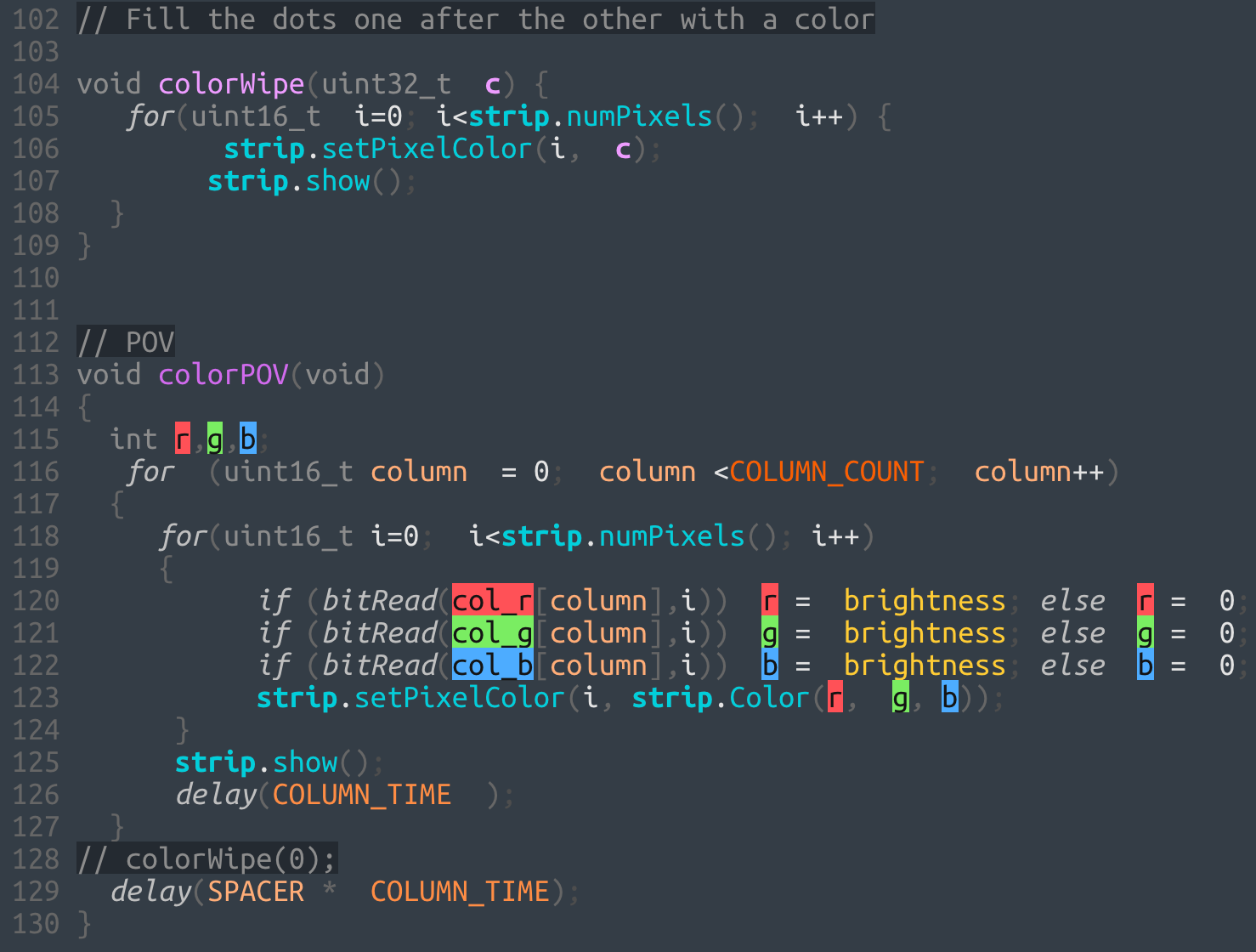
Comments are mixed in with code as constant visual interruptions.
Comments are helpful in that they bring real English to code. They can describe structure. They can explain obscure syntax or clarify what a function is doing. However, they also add clutter and increase the overall length of the document so less of it fits on one screen. (I've been told that bugs increase accordingly.) So there's a constant balancing act between too few (obscure) and too much (obscures the code itself).
Possible solution:
- Comments could be put in the same place where you'd find comments on design or text documents: The sidebar.
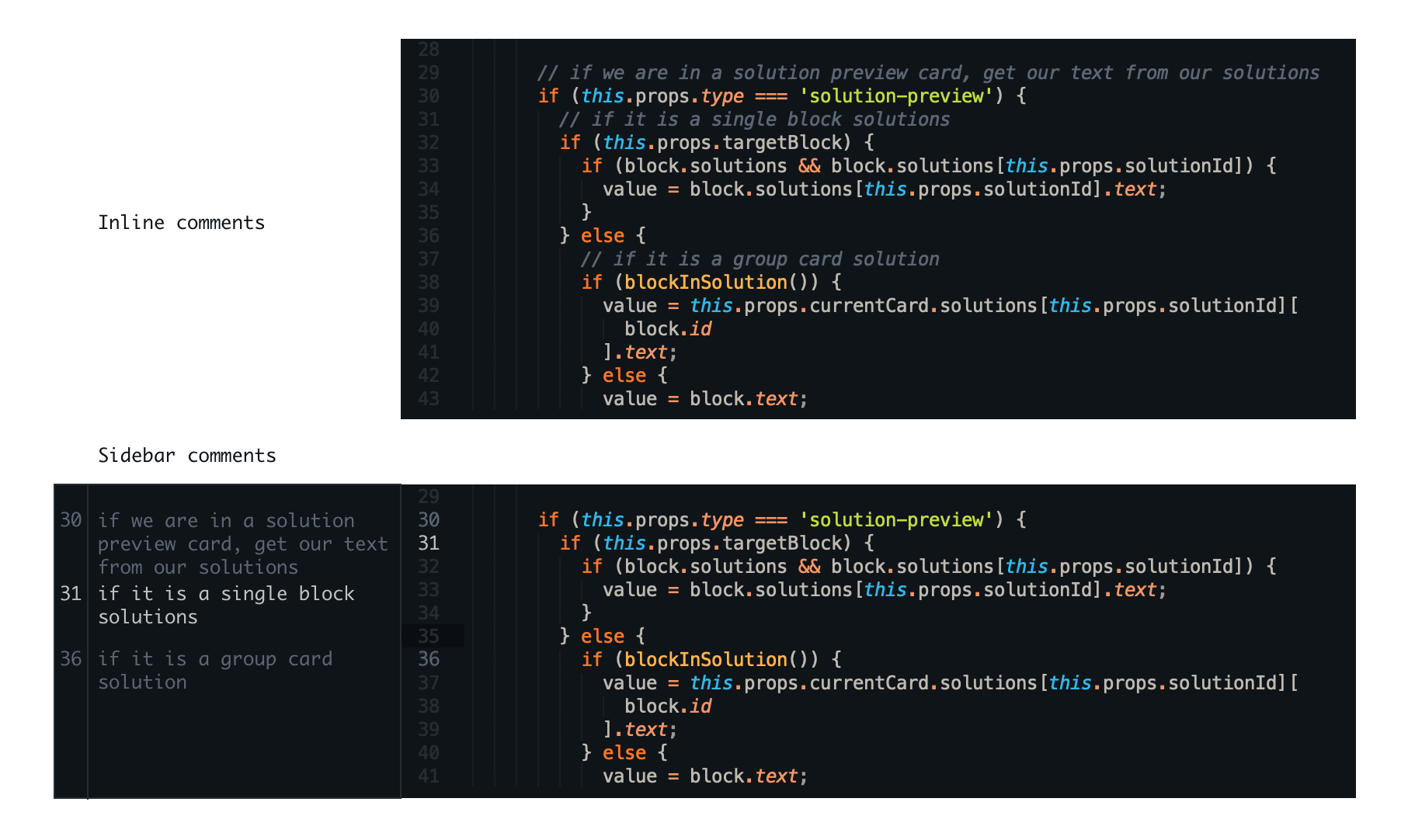
BONUS: Code is typed linearly from top to bottom, yet it can generate asynchronous behavior.
Enemies in a game may walk back and forth and interact WHILE the player character is doing something else entirely. Yet the document that describes all this is something a human tends to read from top to bottom. It's hard to see like the computer, looping one function while, from a human point of view, simultaneously running others. This is something you get used to eventually, but can be a roadblock for beginners.
Solution starting point:
This has been addressed quite extensively by Bret Victor in his Learnable Programming post. The examples in the post are visual, and these are the easiest kinds of programs to "see". It's harder to follow programs that have more abstract processes like getting and manipulating data, although even in those cases it might be helpful to be able to "play" the code frame-by-frame and see what lines are read in what order.
And then?
Perhaps there are issues described here already addressed in various IDEs or languages. I would love to hear about them. (It's really nice to be able to check ideas off a to-do list because they already exist.)
I have met devs who tell me you just start remembering all of this as you code more, and you simply get used to it. Every one of them was a highly specialized full-time dev.
However, there is a growing population of occasional coders; people who don't work full-time with code, or with only one language, or only on one tightly organized megaproject. They have the following things in common:
- They are advanced beginners or intermediates, not experts.
- They are language generalists who remember that it's possible to do a certain thing with a certain library but may frequently forget the syntax or relevant naming.
- They are probably experts in a field outside CS (like design) and therefore have no reason to dedicate their lives to code specifically. Their approach is slapdash and pragmatic in a way that probably horrifies experts but gets stuff done well enough for the purpose.
- They often return to projects left untouched for months and need to remember what that code was doing.
- They reuse and modify a lot of random code found on the internet, which is unlikely to be up to the strict standards found in major software companies.
- They probably don't rely a lot on frameworks or version control.
These people may need to throw together a simple script for a demo every six months, during which time many details and quirks of the language and libraries they're using are likely to evaporate from memory. They don't need features like keyboard shortcuts pro coders use, because their goal is not to produce a lot of code, but rather some very specific, probably pretty simple code, to be reminded of the details that are easily forgotten, and to just make it work.
It doesn't help to tell these people "you should learn to code properly." They have other things to work on. You don't tell a chef wanting to build a doghouse that they should study architecture first, or expect them to model it with the same pro CAD software you use. You let them sketch on paper and use basic hand tools for a result that does what it needs to do.
Would be interesting to develop and test out some of these ideas in practice and see how far one might go. If you have thoughts on any of the above, let me know.